Run Actions from RunJS query
ToolJet allows you to execute various actions within RunJS queries. This guide outlines the syntax and examples for each action.
Run Query Action
Syntax:
queries.queryName.run();
or
await actions.runQuery('queryName');
Example:
In the following screenshot, we demonstrate triggering two different queries, getCustomers
and updateCustomers
, using the two available syntax options for the Run Query
action.
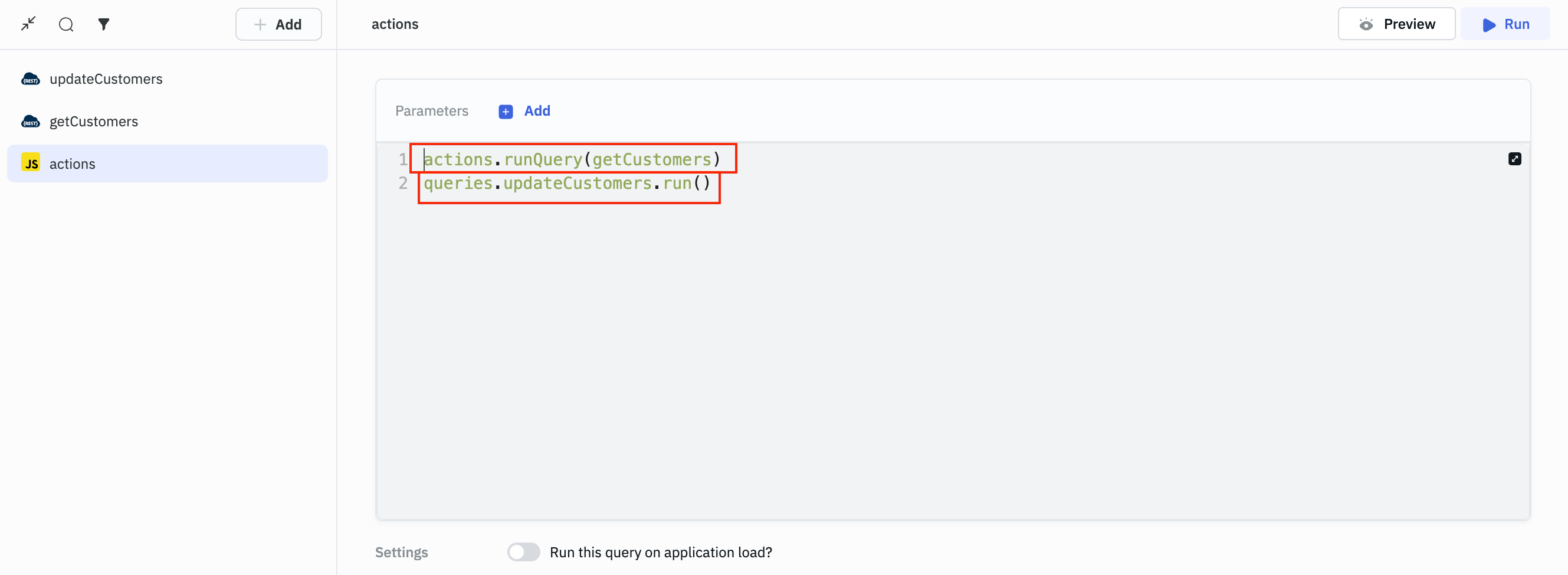
Set Variable Action
Syntax:
actions.setVariable(variableName, variableValue);
Example:
In this example, we set two variables, test
and test2
. Note that test
contains a numerical value, so it is not wrapped in quotes, while test2
is a string and is wrapped in quotes.
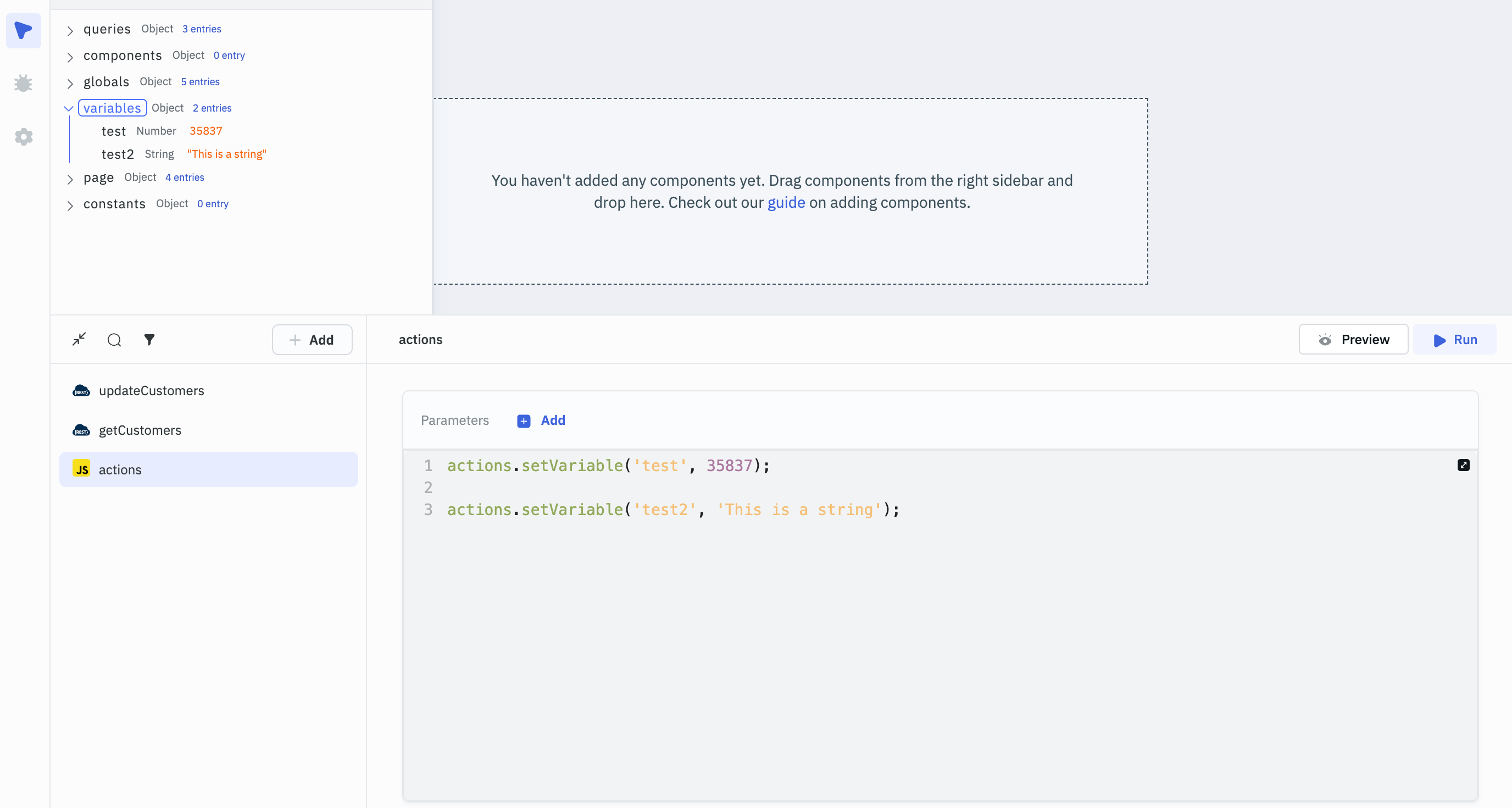
Unset Variable Action
Syntax:
actions.unSetVariable(variableName);
Example:
In the following screenshot, we unset the variable test2
that was created in the previous step.
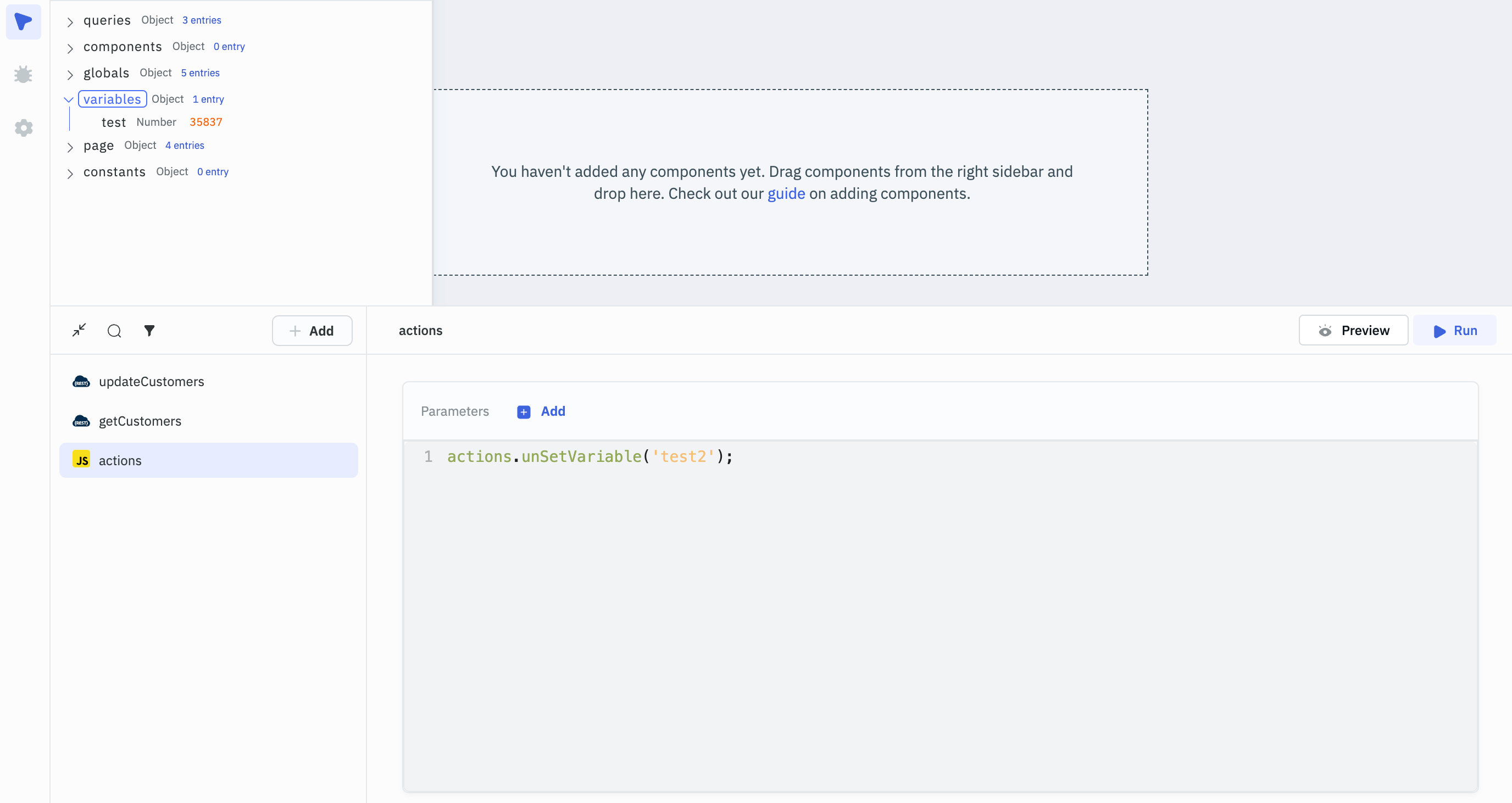
Logout Action
Syntax:
actions.logout();
Example:
Executing actions.logout()
will log out the current user from ToolJet and redirect to the sign-in page.
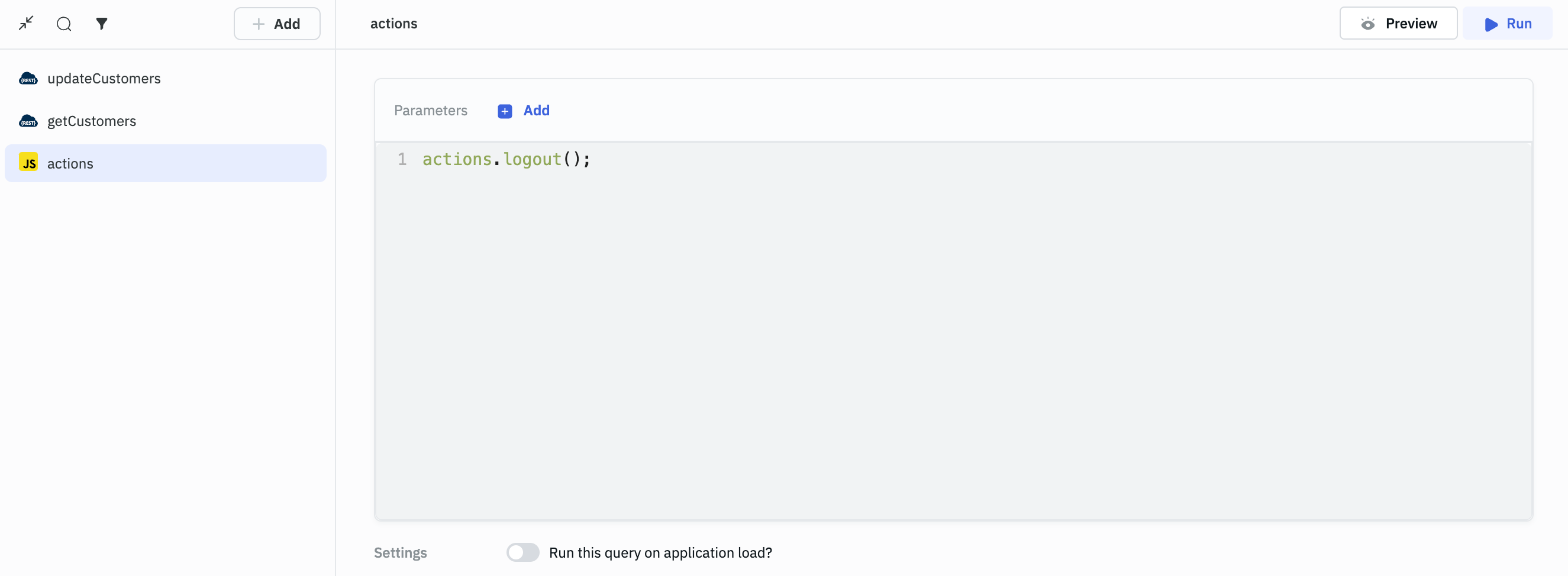
Show Modal Action
Syntax:
actions.showModal('modalName');
Example:
In this example, a modal named formModal
is present on the canvas, and we use a RunJS query to show the modal.
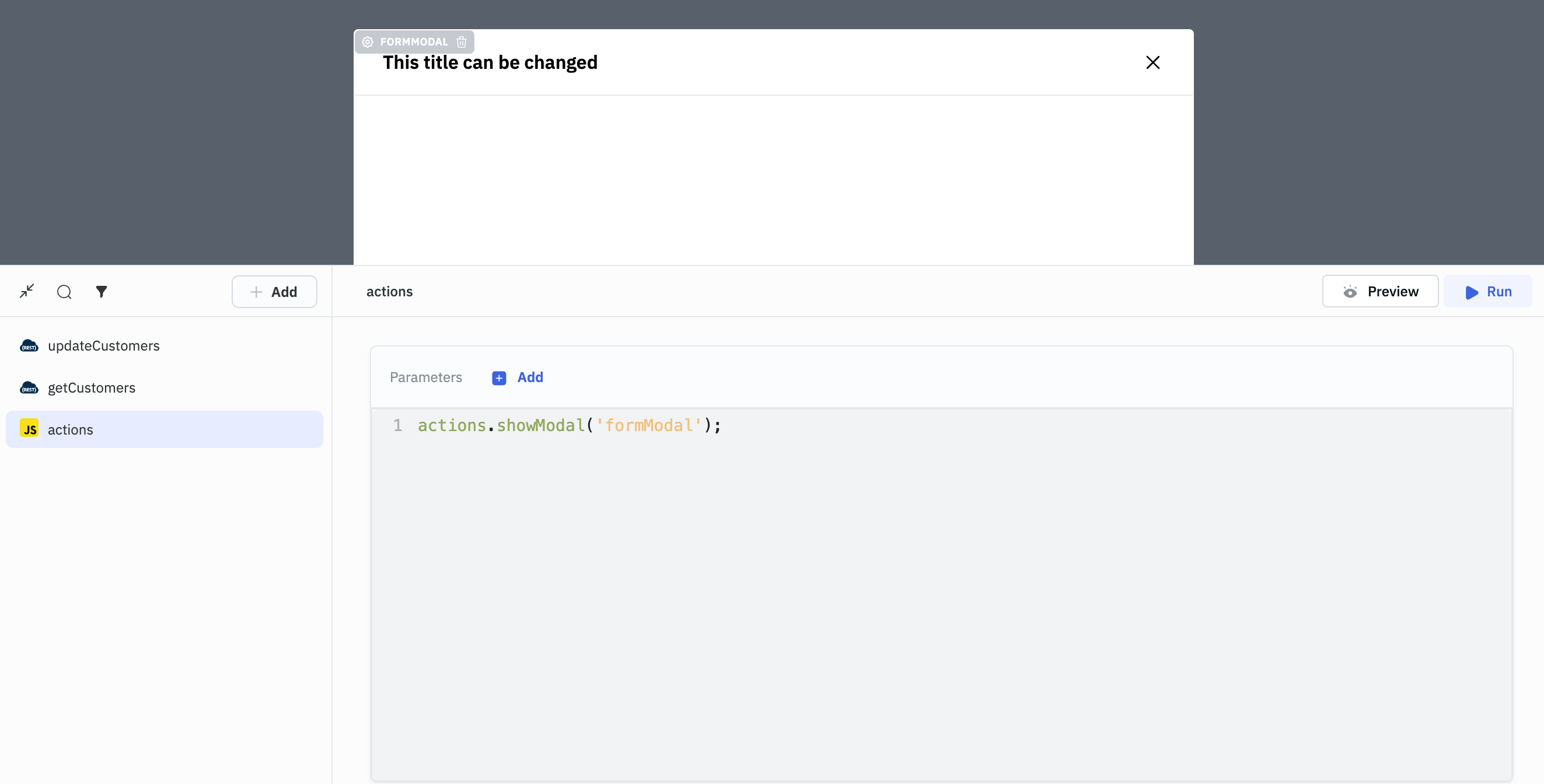
Close Modal Action
Syntax:
actions.closeModal('modalName');
Example:
Here, we use a RunJS query to close the modal that was shown in the previous step.
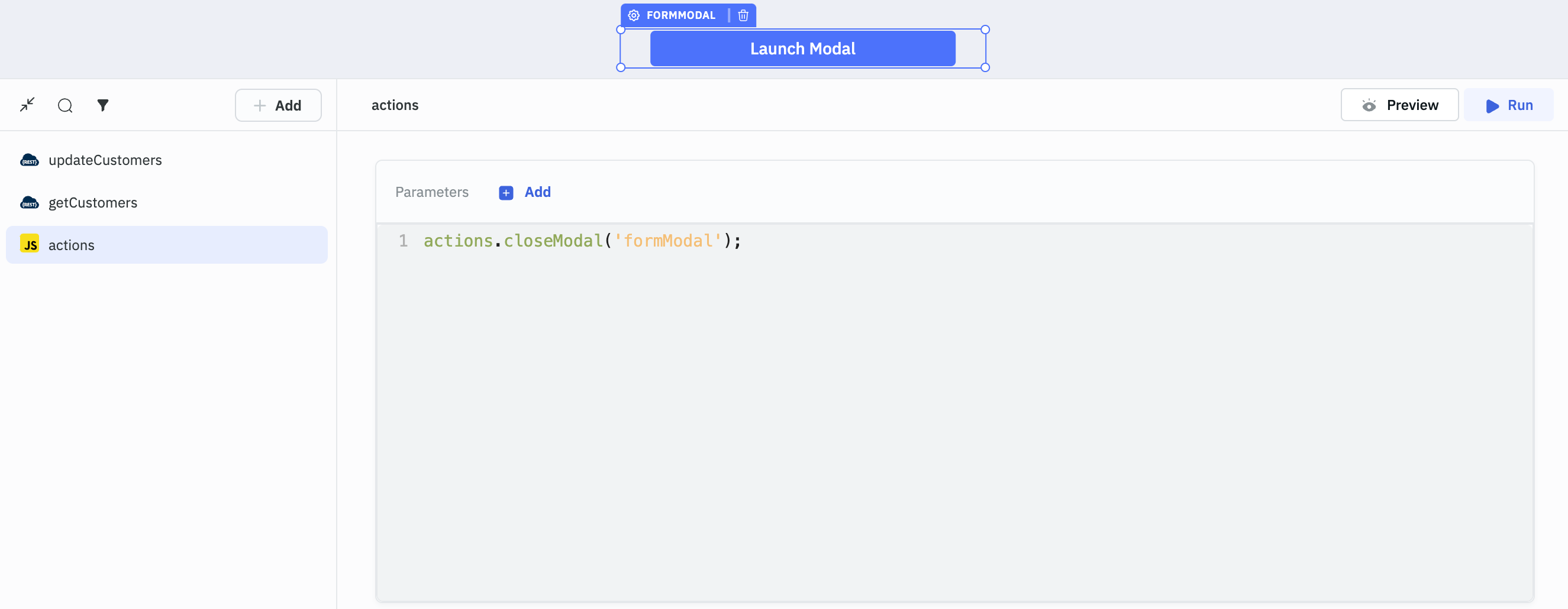
Generate File Action
Syntax:
actions.generateFile('fileName', 'fileType', 'data');
Example for generating a CSV file:
actions.generateFile('csvfile1', 'csv', '{{components.table1.currentPageData}}')
Example for generating a Text file:
actions.generateFile('textfile1', 'plaintext', '{{JSON.stringify(components.table1.currentPageData)}}');
Example for generating a PDF file:
actions.generateFile('Pdffile1', 'pdf', '{{components.table1.currentPageData}}');
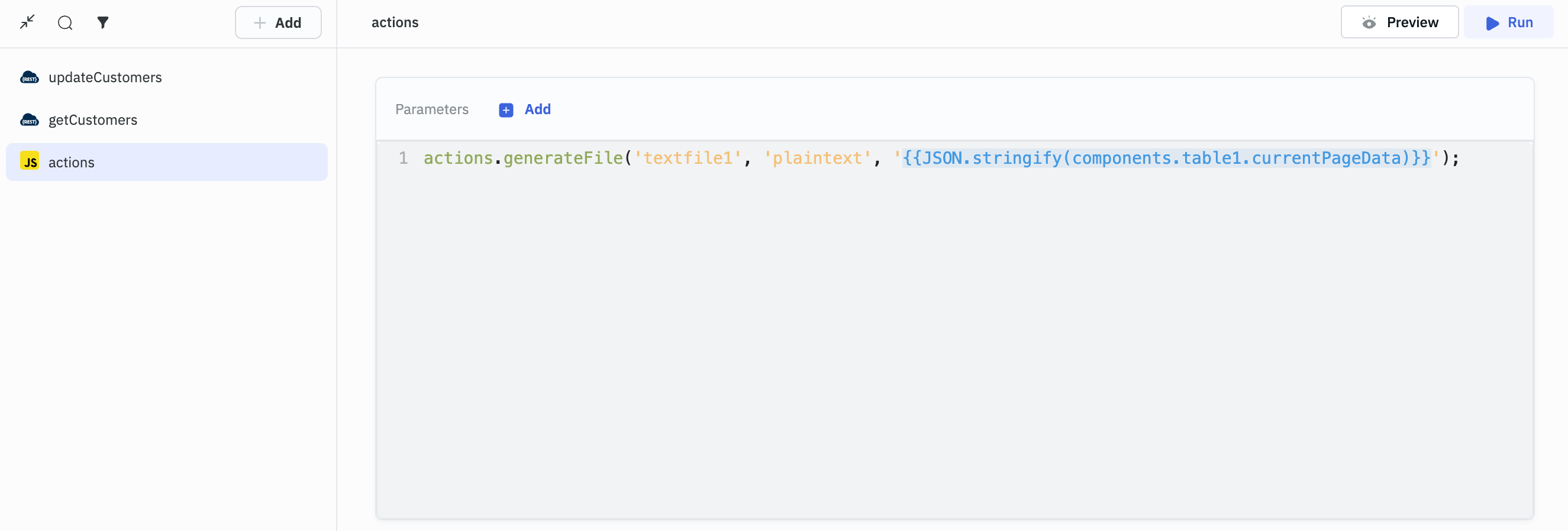
Go to App Action
Syntax:
actions.goToApp('slug', queryparams)
slug
can be found in the URL of the released app after theapplication/
, or in theShare
modal. You can also set a custom slug for the app in theShare
modal or from the global settings in the app builder.queryparams
can be provided like this[{"key":"value"}, {"key2":"value2"}]
.- Only the apps that are released can be accessed using this action.
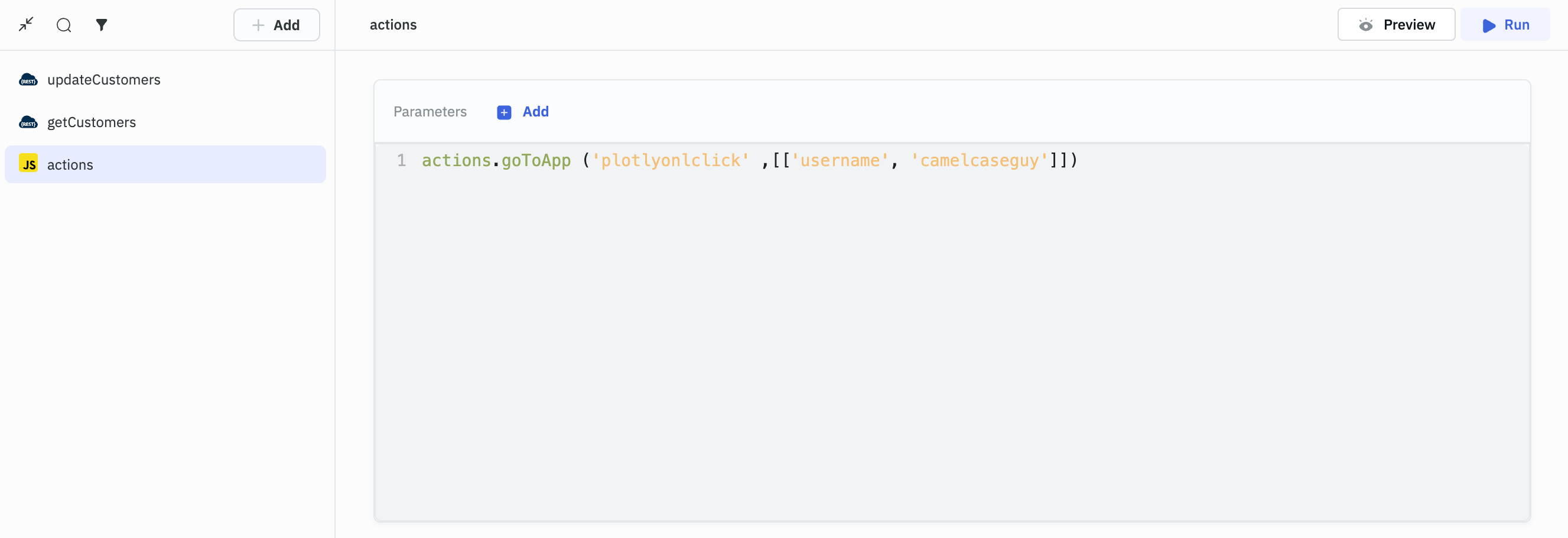
Show Alert Action
Syntax:
actions.showAlert(alertType, message); // alert types are info, success, warning, and error
Example:
actions.showAlert('error', 'This is an error')
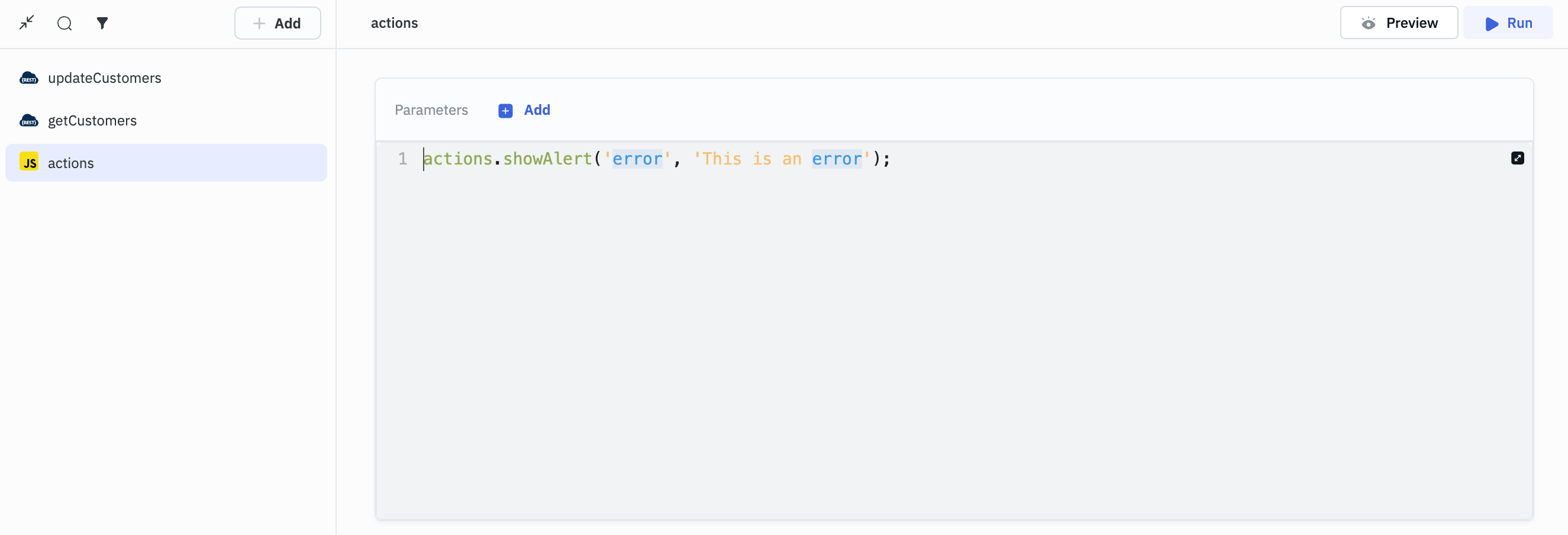
Run Multiple Actions from RunJS Query
To run multiple actions from a RunJS query, use async-await in the function. Here's an example code snippet for running queries and showing an alert at specific intervals:
actions.setVariable('interval', setInterval(countdown, 5000));
async function countdown() {
await queries.restapi1.run();
await queries.restapi2.run();
await actions.showAlert('info', 'This is an information');
}
Actions on pages
Switch page
To switch to a page from the JavaScript query, use the following syntax:
await actions.switchPage('<page-handle>')
Switch page with query parameters
Query parameters can be passed through action such as Switch Page. The parameters are appended to the end of the application URL and are preceded by a question mark (?). Multiple parameters are separated by an ampersand (&).
To switch to a page with query parameters from the JavaScript query, use the following syntax:
actions.switchPage('<pageHandle>', [['param1', 'value1'], ['param2', 'value2']])
Set page variable
Page variables are restricted to the page where they are created and cannot be accessed throughout the entire application like regular variables.
To set a page variable from the JavaScript query, use the following syntax:
await actions.setPageVariable('<variablekey>',<variablevalue>)
This enhanced guide provides a detailed walkthrough of executing various ToolJet actions from RunJS queries.